Introduction
In the contemporary digital landscape, efficiently managing a company’s online presence is crucial for success. The Google My Business API serves as a vital resource that can significantly enhance the management of businesses’ online profiles. This sophisticated application programming interface (API) allows users to automate tasks such as publishing updates, monitoring visibility and reviews, and claiming client listings. In this article, we provide an in-depth guide on utilizing the Google My Business API specifically in C#, accompanied by an illustrative example. Our aim is to equip you with the knowledge and skills necessary to streamline your operations and optimize the management of your online presence using the C# programming language. You can download the pre-built source code here!
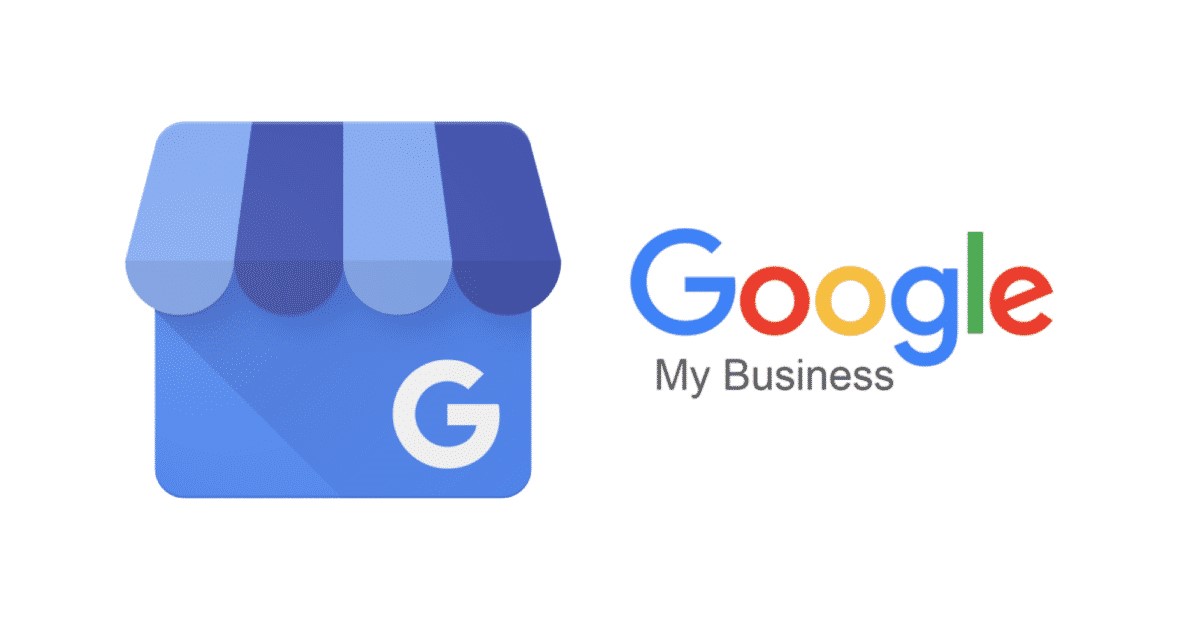
Step 0: Request access to the Google My Business API
Before diving into the implementation of the Google My Business API in C#, it is essential to first obtain access to the API. To do so, you must log in to the Google Cloud Console using the same credentials as your Google My Business account, create a new project, and submit an API activation request form. Generally, the approval process takes 2-3 business days, during which you may be asked to confirm your request or provide additional information. Once your access is granted, you can proceed to configure the API, enable the necessary Google My Business modules, and create an OAuth API key. For a more detailed explanation on requesting access to the Google My Business API, please refer to our previous article.
Step 1: Set Your Google Client ID, Secret, and Scopes
Before using the Google My Business API, prepare your Google Client ID, Secret, and desired scopes. These can be obtained through the Google Cloud Console after requesting access to the API. Replace the placeholders in the example code with your actual Google Client ID and Secret:
string googleClientId = "your-google-client-id-goes-here";
string googleClientSecret = "your-google-client-secret-goes-here";
string[] scopes = { "https://www.googleapis.com/auth/plus.business.manage" };
Step 2: Authenticate Yourself to the Google My Business API
On first run, the program shows an authentication window, and the machine stores the authentication token. Subsequent authentications will not require a login unless you decide to update your scopes.
UserCredential credential = GoogleAuthenticationHandler.Authenticate(googleClientId, googleClientSecret, scopes);
Step 3: Access Google My Business Account and Locations
Ensure that your account has the authorization to use the Google My Business API. With access, utilize the code snippet to access your Google My Business account and related locations. This code will display the Google My Business account name and the associated locations.
using (var gmbAccountService = new MyBusinessAccountManagementService(new BaseClientService.Initializer() { HttpClientInitializer = credential }))
{
var accounts = gmbAccountService.Accounts.List().Execute();
foreach (var account in accounts.Accounts)
{
Console.WriteLine(account.Name);
Console.WriteLine("\nListing managed locations for this account:");
using (var gmbLocationService = new MyBusinessBusinessInformationService(new BaseClientService.Initializer() {HttpClientInitializer = credential}))
{
var locationsRequest = gmbLocationService.Accounts.Locations.List(account.Name);
locationsRequest.ReadMask = "name";
var locations = locationsRequest.Execute();
foreach (var location in locations.Locations)
Console.WriteLine(location.Name);
}
}
Console.ReadLine();
}
Conclusion
The Google My Business API offers a variety of features that can help you manage your business listings more effectively. With the example code provided, you can easily access your Google My Business account and associated locations. Remember to refer to the Google API documentation for more information on the available methods and functionalities.